What is Mock Server?
Mock Server is a considerably useful tool when testing integrations against HTTP services. It is especially useful for integration tests. With Mock Server, it is possible to launch an HTTP server within our test suite and define how it should behave when receiving specific requests from a client application.
Additionally, Mock Server is able to verify that the client is making the expected requests when a particular execution flow is being executed.
In what scenarios is Mock Server useful?
- API First Development: If you are using the API First development methodology, Mock Server can be helpful to quickly have a backend application to integrate without needing any real application developed.
- Isolation: Integration tests will be completely isolated from a pre-production or integration environment. This brings two additional benefits: it adds reproducibility to our tests and simplifies their setup by removing external dependencies.
- Execution Speed: Tests will run faster as they do not depend on the network or external services.
Deploying Mock Server as a Docker container
Mock Server can be deployed as a Java application in a Docker container. This can be achieved with the following docker-compose:
services:
mockServer:
image: mockserver/mockserver:5.14.0
ports:
- 12345:1080
environment:
MOCKSERVER_WATCH_INITIALIZATION_JSON: "true"
MOCKSERVER_PROPERTY_FILE: /config/mockserver.properties
MOCKSERVER_INITIALIZATION_JSON_PATH: /config/initializerJson.json
volumes:
- type: bind
source: .
target: /config
Running this docker-compose with the command docker-compose up
will create a Docker container accessible at the URL http://localhost:12345.
To define the expected behavior of the external HTTP server, there are two preferred options: either use the Mock Server REST API or a JSON initialization file.
If you are integrating a service and you know the expected responses for specific inputs or want to test specific scenarios, you can define various request matchers, such as:
- Using the HTTP request path.
- Using a matcher with the HTTP request body expecting a JSON.
- Using a matcher with the HTTP request body matching a regular expression.
- Using a matcher for the HTTP request headers.
If you want to get into the details, check out this GitHub repository, which contains an example of Mock Server deployed with Docker.
You can download the repository and deploy it with docker-compose up
.
Once the Docker container is ready, access http://localhost:12345/mockserver/dashboard to view the created expectations and the state of Mock Server.
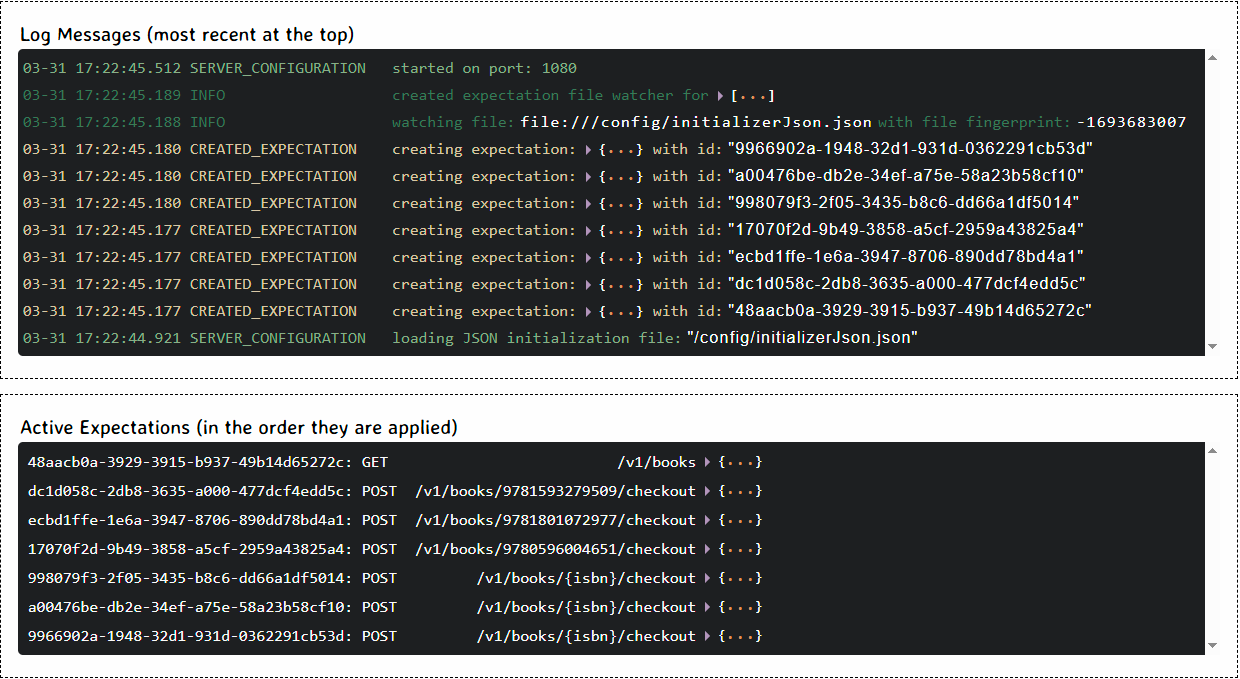
MockServer Dashboard
Integration Tests in Java with Mock Server
Installation and Configuration
To start using Mock Server in your Java project, you will need to add the necessary dependencies to your build file.
If you are using Gradle, add the following lines to your build.gradle
file:
testImplementation 'org.mock-server:mockserver-netty:5.11.2'
testImplementation 'org.mock-server:mockserver-client-java:5.11.2'
Basic Example
Let’s look at an example use case: a middleware library to a REST API for managing book reservations. The library will have a method to retrieve available books and another method to reserve a book.
Using Mock Server, we can mock an HTTP server directly from our tests. To initialize it, simply invoke the ClientAndServer.startClientAndServer(<port>)
method before each test.
class LibraryClientTestImpl {
private static final int DEFAULT_PORT = 8181;
private final LibraryClient libraryClient = new LibraryClientImpl(
new LibraryClientConfig()
.setBaseUrl("http://localhost:" + DEFAULT_PORT)
.setTimeout(1000)
);
private ClientAndServer clientAndServer;
@BeforeEach
void setUp() {
// Start a Mock Server
clientAndServer = ClientAndServer.startClientAndServer(DEFAULT_PORT);
}
@AfterEach
void tearDown() {
// Stop the Mock Server
clientAndServer.stop();
}
@Test
void testGetBooks() {
final String responseBody = "[{"
+ " \"isbn\": \"9781593279509\", "
+ " \"title\":\"Eloquent Javascript\", "
+ " \"author\":\"Marijin Haverbeke\""
+ "}, {"
+ " \"isbn\": \"9781801072977\", "
+ " \"title\":\"Microservices with Spring Boot and Spring Cloud\", "
+ " \"author\":\"Magnus Larsson\" "
+ "}, {"
+ " \"isbn\": \"9780596004651\", "
+ " \"title\":\"Head First Java\", "
+ " \"author\":\"Kathy Sierra\""
+ "}]";
// Define an expectation for when a client makes a GET request to the /v1/books endpoint
clientAndServer.when(HttpRequest.request()
.withMethod("GET")
.withPath("/v1/books"))
.respond(
HttpResponse.response()
.withStatusCode(200)
.withHeader("Content-Type", "application/json")
.withBody(responseBody)
);
// Trigger the request through the library client to test it
List<Book> books = libraryClient.getBooks();
Book book;
Assertions.assertEquals(3, books.size());
// Verify the first book
book = books.get(0);
Assertions.assertEquals("9781593279509", book.getIsbn());
Assertions.assertEquals("Eloquent Javascript", book.getTitle());
Assertions.assertEquals("Marijin Haverbeke", book.getAuthor());
// Verify the remaining books...
// Verify that Mock Server received the GET /v1/books request exactly once.
RequestDefinition[] requests =
clientAndServer.retrieveRecordedRequests(HttpRequest.request()
.withMethod("GET")
.withPath("/v1/books"));
Assertions.assertEquals(1, requests.length);
}
// ...
In the test, you can see how an expectation is defined programmatically, similar to how we would create a stub in a unit test. Once the expectation is configured, the request is executed to retrieve the available books from the API, and the received data is verified against the expected results defined in the stub.
As an extra step, we also validate that the request was made to Mock Server and that the request was executed only once.
Get started with Mock Server
Mock Server is a very useful tool for validating scenarios that may occur when integrating with a third-party API. It is a tool worth considering when an application needs to ensure proper integration with a third-party API, adding quality and coverage to ensure that the contract is fulfilled in every build of the application.